Splitting Strings to Characters for Sequential Effects and Character Animation
Splitting the string(contains div) into chars(of span) using javascript to achieve sequential effects and character animation.
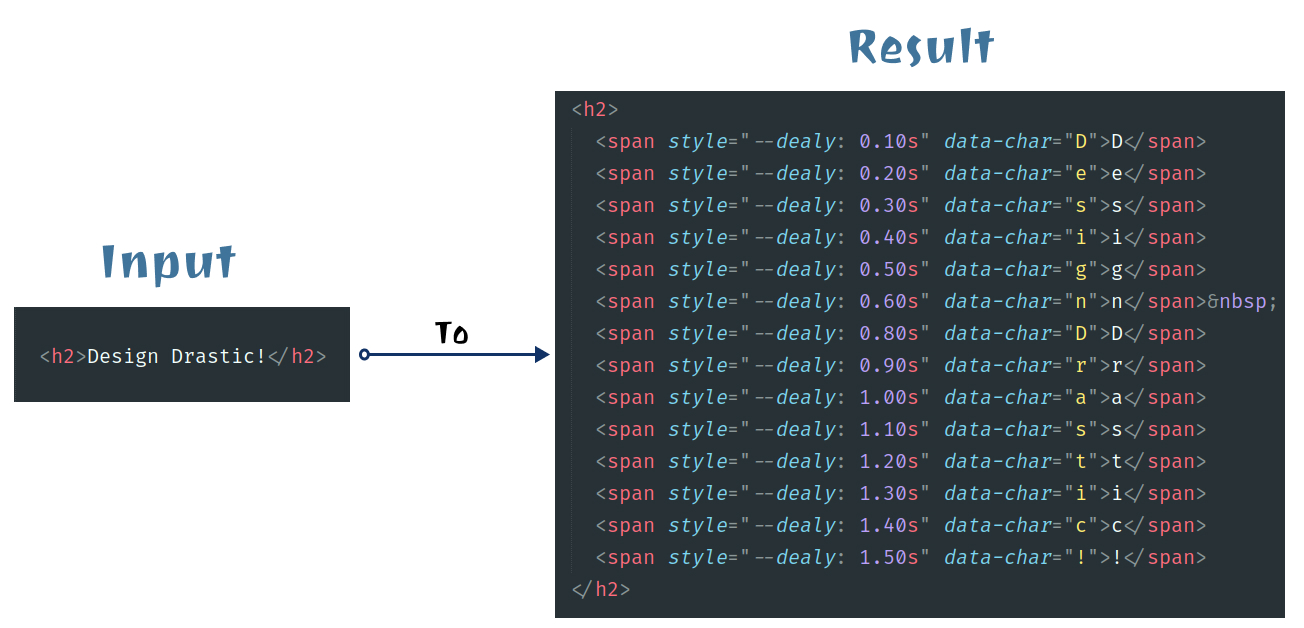
In this tutorial we will split/convert the string into chars and apply the required attributes(data attribute) that will use to animate chars one by one. Input
For our demonstration, let’s consider a simple heading enclosed within a <h2>
tag:
<h2>Design Drastic!</h2>
Output
Output
Our goal is to convert each character within the heading into a separate <span>
element, each with a distinct animation delay. Here’s what the transformed HTML will look like:
<h2>
<span style="--dealy: 0.10s" data-char="D">D</span>
<span style="--dealy: 0.20s" data-char="e">e</span>
<span style="--dealy: 0.30s" data-char="s">s</span>
<span style="--dealy: 0.40s" data-char="i">i</span>
<span style="--dealy: 0.50s" data-char="g">g</span>
<span style="--dealy: 0.60s" data-char="n">n</span>
<span style="--dealy: 0.80s" data-char="D">D</span>
<span style="--dealy: 0.90s" data-char="r">r</span>
<span style="--dealy: 1.00s" data-char="a">a</span>
<span style="--dealy: 1.10s" data-char="s">s</span>
<span style="--dealy: 1.20s" data-char="t">t</span>
<span style="--dealy: 1.30s" data-char="i">i</span>
<span style="--dealy: 1.40s" data-char="c">c</span>
<span style="--dealy: 1.50s" data-char="!">!</span>
</h2>
Implementation
Let’s break down the process of achieving this captivating animation effect using JavaScript.
Get the String Content: We begin by selecting the <h2>
element and obtaining its text content.
let str = document.querySelector("h2");
Split Text into Characters: The string is then split into an array of individual characters.
let title = str.innerText.split("");
Create Character Elements: We iterate through the character array, creating a <span>
element for each character. Special cases for newline characters and spaces are also considered.
let span = "";
let delay = 0.1;
title.forEach((char) => {
if (char == "\n") {
span += '<div class="clearfix"></div>';
} else if (char == " ") {
span += " ";
} else {
span +=
'<span style="--dealy: ' +
delay.toFixed(2) +
's" data-char="' +
char +
'">' +
char +
"</span>";
}
delay += 0.1;
});
Replace Content with Animated Elements: Finally, we replace the content of the <h2>
element with the generated <span> elements, each with its animation delay and character data.
str.innerHTML = span;
Explaination:
In the provided code snippet, the process is comprehensively detailed:
- The <h2> element is obtained using document.querySelector.
- The text content of the heading is split into an array of characters.
- As we iterate through the character array, each character is encapsulated within a
<span>
element. The custom attribute —delay controls the animation timing. - Newline characters are replaced with a clearfix
<div>
, while spaces are replaced with non-breaking space entities for correct rendering. - The modified HTML content, containing animated
<span>
elements, is assigned back to the<h2>
element.
With this step-by-step guide, you can now convert your string into set of chars to animate further.
Thank you for taking the time to read our tutorial! If you found this guide helpful and insightful, we encourage you to share it with others!