Retrieving Input Type Color Values using Javascript OnChange Event
Retrieve the input type color value immediately when user will change the color using native color picker.
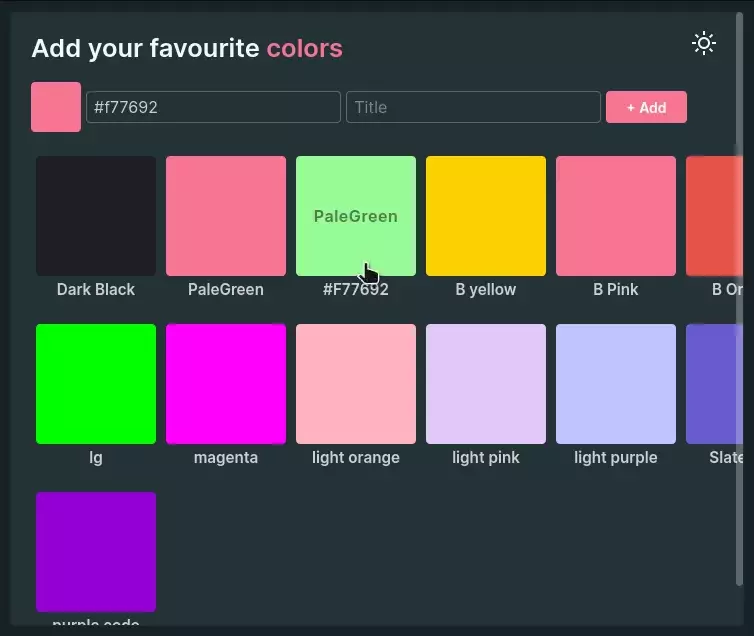
In this article, we’ll instantly capture color changes(hexcode value) from native color picker using javascript onInput event.
To promptly capture color changes made by users using the native color picker, you can leverage the input event. Unlike the onchange event, which may require additional actions to trigger, the input event responds instantaneously as the user interacts with the native color picker. Let’s explore how to achieve this.
Html syntax
<input type="color" id="color" />
Javascript
- Retrieve the color input element using its ID:
let color = document.getElementById("color");
Attach an input event listener to the color input element:
With the input event listener in place, the selected color value will be promptly logged to the console each time the user interacts with the color picker.
oninput event occurs when the text content of an element is changed through the user interface. onchange occurs when checked state, selection or content of an element changed by the user.
input event js
color.addEventListener('input', function(e) {
console.log(this.value);
}
The above snippet will return the value of the color when user drag in the input type color box immediately.
Demo
Chrome
Firefox
The demo code HTML
<input type="color" id="color" />
<input type="text" id="clr_input" value="#000000" />
<label id="clr_lbl">#000000</label> <span id="clr_span"></span>
Javascript
let color = document.getElementById("color");
color.addEventListener("input", function (e) {
clr_lbl.innerHTML = this.value;
clr_input.value = this.value;
clr_lbl.style.color = this.value;
clr_span.style.background = this.value;
});
We’ll implement same logic for Vue & React apps.
Vue js Set the v-model and data
data: {
return { color: "" };
}
Next, create the input color tag
<input id="color" v-model="color" @input="handleChange" type="color" />
In above snippet we will use the method handleChange onInput event listener. We will use @input or v-on:input to trigger the event. Vue js method to reflect the color change values.
methods: { handleChange() { console.log(this.color); } }
React js In reacts js we’ll first set the color to state
constructor() {
this.state = {color: ''}
}
Hml
<input type="text" value="{this.state.color}" onChange="{this.handleChange}" />
React method to handle the change event.
handleChange(event) { this.setState({color: event.target.color}); }
By creating the input event, you ensure that color changes are swiftly captured from the native color picker immediately.
Thanks for reading!